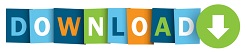

# Those in single quotes are normal strings, like 'Peter' # Notice the type of quotes - important ! # Invoke-Expression $command | Tee-Object -Variable cmdOutput # or if you need also output to a variable # all passed-in entries into 1 single string line Works well: # function with one argument will flatten This directive is specifically designed to convert a string to runnable command. That flattens them, including arrays, to a single string, which I then execute using PS's 'Invoke-Expression'. Then I use a simple trick of passing all arguments inside double quotes to a function with 1 single parameter. This way it is very easy to read and edit. I place arguments in an array, 1 per line. I use this simple, clean and effective method. References : Powershell/Scripting/Start-Process
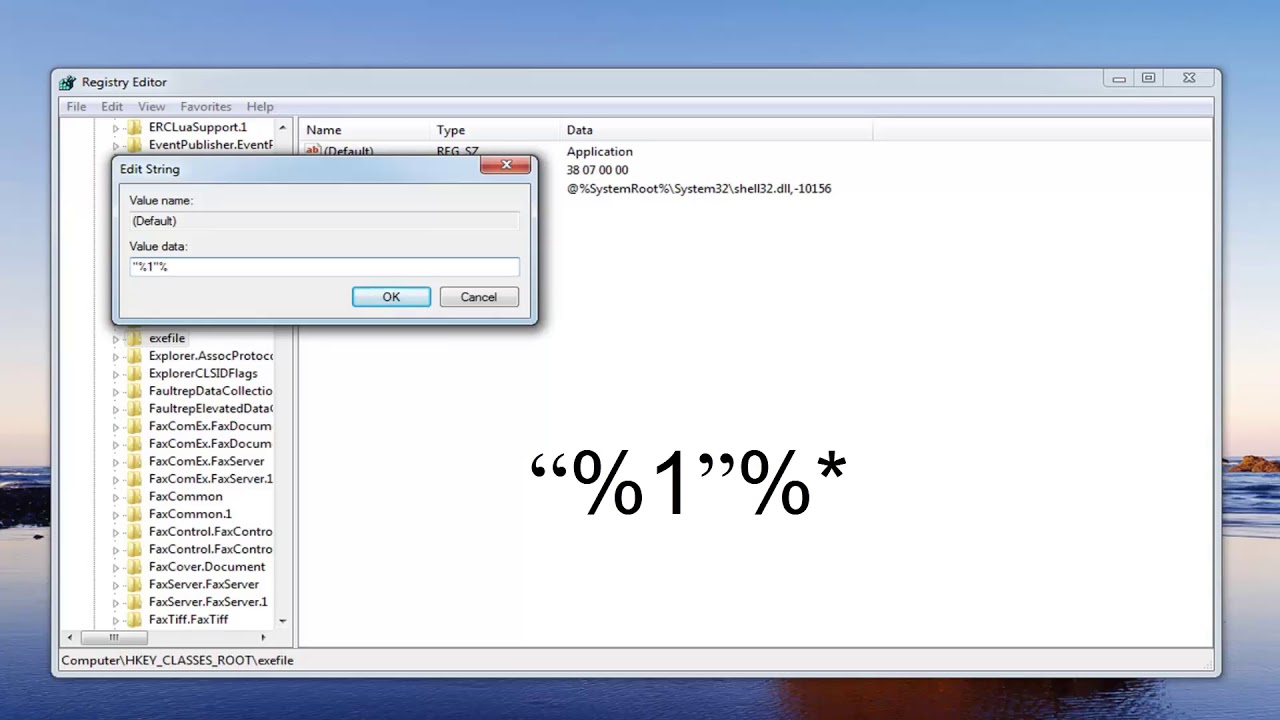
By default Windows PowerShell opens a new window. Parameter is used to display the new process in the current console window. Note that in powershell to represent the quotation mark ( " ) in a string you should insert the grave accent ( ` ) (This is the key above the Tab key in the US keyboard). This method is easier as it allows to type your parameters in one go. In your case Start-Process -NoNewWindow -FilePath "C:\Program Files\IIS\Microsoft Web Deploy\msdeploy.exe" -ArgumentList "-verb:sync -source:dbfullsql=`"Data Source=mysource Integrated Security=false User ID=sa Pwd=sapass! Database=mydb `" -dest:dbfullsql=`"Data Source=.\mydestsource Integrated Security=false User ID=sa Pwd=sapass! Database=mydb `",computername=10.10.10.10,username=administrator,password=adminpass" Simple Example Start-Process -NoNewWindow -FilePath "C:\wamp64\bin\mysql\mysql5.7.19\bin\mysql" -ArgumentList "-u root -proot -h localhost" In this method you separate each and every parameter in the ArgumentList using commas. In your case Start-Process -NoNewWindow -FilePath "C:\Program Files\IIS\Microsoft Web Deploy\msdeploy.exe" -ArgumentList "-verb:sync","-source:dbfullsql=`"Data Source=mysource Integrated Security=false User ID=sa Pwd=sapass! Database=mydb `"","-dest:dbfullsql=`"Data Source=.\mydestsource Integrated Security=false User ID=sa Pwd=sapass! Database=mydb `"","computername=10.10.10.10","username=administrator","password=adminpass" Microsoft recommends using Start-Process.Ī simple example Start-Process -NoNewWindow -FilePath "C:\wamp64\bin\mysql\mysql5.7.19\bin\mysql" -ArgumentList "-u root","-proot","-h localhost" There are other methods like using the Call Operator ( &), Invoke-Expression cmdlet etc. There are quite a few methods you can use to do it.
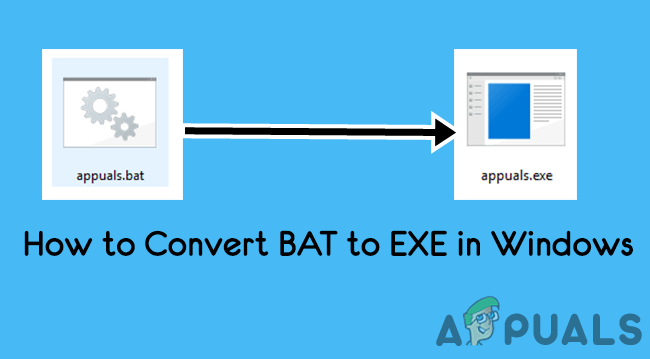
#What program runs exe files windows 10 update#
UPDATE : This situation gets much easier to handle in PowerShell V3.
#What program runs exe files windows 10 series#
If this is an area of pain for you, then please vote up this PowerShell bug submission.įor more information on how PowerShell parses, check out my Effective PowerShell blog series - specifically item 10 - "Understanding PowerShell Parsing Modes"

:-) They also realize this is an area of pain, but they are driven by the number of folks are affected by a particular issue. They were quite helpful in showing me the specific incantation of single & double quotes to get the desired result - if you needed to keep the internal double quotes in place. Apparently that isn't necessary because even cmd.exe will strip those out.īTW, hats off to the PowerShell team. It turns out I was trying too hard before to maintain the double quotes around the connection string. Using echoargs you can experiment until you get it right, for example: PS> echoargs -verb:sync "-source:dbfullsql=Data Source=mysource Integrated Security=false User ID=sa Pwd=sapass! Database=mydb " You just replace the EXE file with echoargs - leaving all the arguments in place, and it will show you how the EXE file will receive the arguments, for example: PS> echoargs -verb:sync -source:dbfullsql="Data Source=mysource Integrated Security=false User ID=sa Pwd=sapass! Database=mydb " -dest:dbfullsql="Data Source=.\mydestsource Integrated Security=false User ID=sa Pwd=sapass! Database=mydb ",computername=10.10.10.10,username=administrator,password=adminpass The PowerShell Community Extensions has such a tool. When you invoke an EXE file like this with complex command line arguments it is usually very helpful to have a tool that will show you how PowerShell sends the arguments to the EXE file. If you want PowerShell to interpret the string as a command name then use the call operator (&) like so: PS> & 'C:\Program Files\IIS\Microsoft Web Deploy\msdeploy.exe'Īfter that you probably only need to quote parameter/argument pairs that contain spaces and/or quotation chars. When PowerShell sees a command starting with a string it just evaluates the string, that is, it typically echos it to the screen, for example: PS> "Hello World"
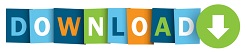